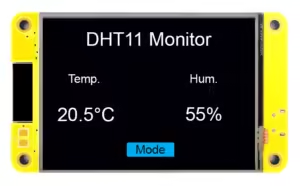
Dans cet article nous allons voir comment afficher sur l’écran ESP32-2432S028 (ESP32 CYD) la température et l’humidité provenant d’un DHT11.
Connecter le DHT11 a l’ESP32 2432S028
Au niveau du connecteur le plus proche du lecteur de carte SD (Voir image ci-dessous) connectez votre DHT11 en suivant le tableau suivant (Pin du connecteur: GND | IO22 | IO27 | 3.3V)
DHT11 | ESP32 CYD / ESP32 2432S028 |
GND | GND |
OUT | GPIO 22 |
VCC | VCC |
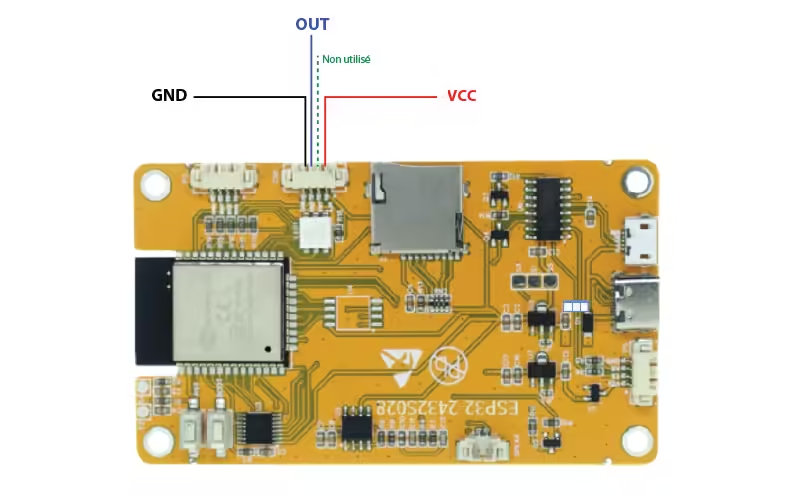
Programmer un ESP32 CYD pour afficher la température et l’humidité d’un DHT11
Pour commencer installez ces deux bibliothèques:
- XPT2046_Touchscreen.h
- DHT.h (DHT sensor library par Adafruit)
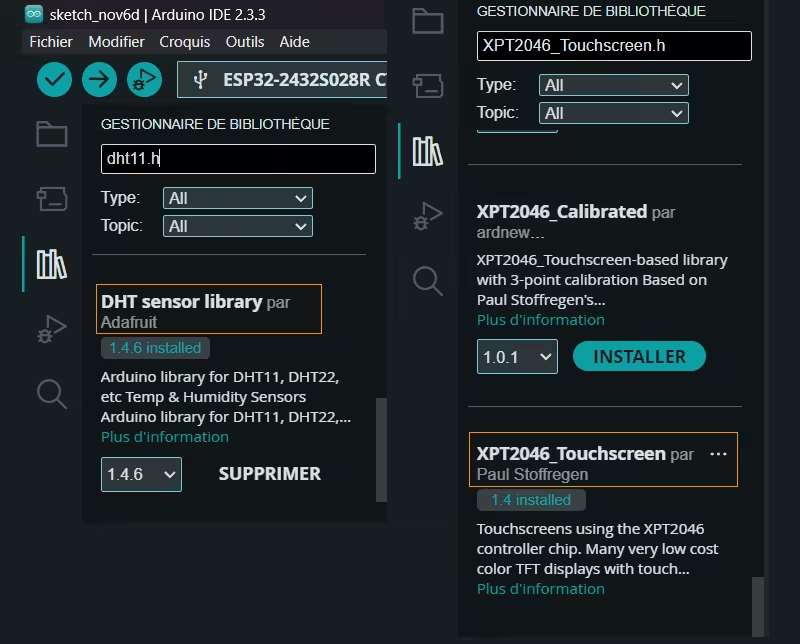
Copiez-collez ensuite ce code dans votre logiciel Arduino et téléversez le.
#include <Arduino.h>
#include <lvgl.h>
#include <TFT_eSPI.h>
#include <XPT2046_Touchscreen.h>
#include <DHT.h>
// Définitions des pins
#define DHTPIN 22
#define DHTTYPE DHT11
#define XPT2046_IRQ 36
#define XPT2046_MOSI 32
#define XPT2046_MISO 39
#define XPT2046_CLK 25
#define XPT2046_CS 33
// Configuration de l'écran
#define SCREEN_WIDTH 240
#define SCREEN_HEIGHT 320
#define DRAW_BUF_SIZE (SCREEN_WIDTH * SCREEN_HEIGHT / 10 * (LV_COLOR_DEPTH / 8))
// Initialisation des objets
SPIClass touchscreenSPI = SPIClass(VSPI);
XPT2046_Touchscreen touchscreen(XPT2046_CS, XPT2046_IRQ);
DHT dht(DHTPIN, DHTTYPE);
// Variables globales
uint32_t draw_buf[DRAW_BUF_SIZE / 4];
int x, y, z;
static lv_obj_t *temp_label;
static lv_obj_t *temp_value_label;
static lv_obj_t *humidity_label;
static lv_obj_t *humidity_value_label;
void touchscreen_read(lv_indev_t * indev, lv_indev_data_t * data) {
if(touchscreen.tirqTouched() && touchscreen.touched()) {
TS_Point p = touchscreen.getPoint();
x = map(p.x, 200, 3700, 1, SCREEN_WIDTH);
y = map(p.y, 240, 3800, 1, SCREEN_HEIGHT);
z = p.z;
data->state = LV_INDEV_STATE_PRESSED;
data->point.x = x;
data->point.y = y;
} else {
data->state = LV_INDEV_STATE_RELEASED;
}
}
void updateDHT11() {
float temperature = dht.readTemperature();
float humidity = dht.readHumidity();
char temp_buf[16];
char hum_buf[16];
if (!isnan(temperature) && !isnan(humidity)) {
snprintf(temp_buf, sizeof(temp_buf), "%.1f°C", temperature);
snprintf(hum_buf, sizeof(hum_buf), "%.0f%%", humidity);
if(temp_value_label != NULL) {
lv_label_set_text(temp_value_label, temp_buf);
}
if(humidity_value_label != NULL) {
lv_label_set_text(humidity_value_label, hum_buf);
}
Serial.printf("Temperature: %.1f°C, Humidite: %.0f%%\n", temperature, humidity);
} else {
if(temp_value_label != NULL) {
lv_label_set_text(temp_value_label, "--°C");
}
if(humidity_value_label != NULL) {
lv_label_set_text(humidity_value_label, "--%");
}
Serial.println("Erreur de lecture DHT11");
}
}
void event_handler_btn(lv_event_t * e) {
lv_event_code_t code = lv_event_get_code(e);
lv_obj_t * obj = (lv_obj_t *)lv_event_get_target(e); // Ajout du cast
if(code == LV_EVENT_VALUE_CHANGED) {
if(lv_obj_has_state(obj, LV_STATE_CHECKED)) {
// Mode clair
lv_obj_set_style_bg_color(lv_screen_active(), lv_color_make(255, 255, 255), LV_PART_MAIN | LV_STATE_DEFAULT);
lv_obj_set_style_text_color(lv_screen_active(), lv_color_make(0, 0, 0), LV_PART_MAIN | LV_STATE_DEFAULT);
} else {
// Mode sombre
lv_obj_set_style_bg_color(lv_screen_active(), lv_color_make(0, 0, 0), LV_PART_MAIN | LV_STATE_DEFAULT);
lv_obj_set_style_text_color(lv_screen_active(), lv_color_make(255, 255, 255), LV_PART_MAIN | LV_STATE_DEFAULT);
}
}
}
void lv_create_main_gui(void) {
// Configuration initiale (mode sombre par défaut)
lv_obj_set_style_bg_color(lv_screen_active(), lv_color_make(0, 0, 0), LV_PART_MAIN | LV_STATE_DEFAULT);
lv_obj_set_style_text_color(lv_screen_active(), lv_color_make(255, 255, 255), LV_PART_MAIN | LV_STATE_DEFAULT);
// Titre
lv_obj_t * title = lv_label_create(lv_screen_active());
lv_obj_set_style_text_font(title, &lv_font_montserrat_20, 0);
lv_label_set_text(title, "DHT11 Monitor");
lv_obj_align(title, LV_ALIGN_TOP_MID, 0, 20);
// Colonne Température
temp_label = lv_label_create(lv_screen_active());
lv_obj_set_style_text_font(temp_label, &lv_font_montserrat_16, 0);
lv_label_set_text(temp_label, LV_SYMBOL_HOME " Temp."); // Changé le symbole
lv_obj_align(temp_label, LV_ALIGN_LEFT_MID, 30, -30);
temp_value_label = lv_label_create(lv_screen_active());
lv_obj_set_style_text_font(temp_value_label, &lv_font_montserrat_30, 0);
lv_label_set_text(temp_value_label, "--°C");
lv_obj_align(temp_value_label, LV_ALIGN_LEFT_MID, 30, 10);
// Colonne Humidité
humidity_label = lv_label_create(lv_screen_active());
lv_obj_set_style_text_font(humidity_label, &lv_font_montserrat_16, 0);
lv_label_set_text(humidity_label, LV_SYMBOL_TINT " Hum."); // Changé le symbole
lv_obj_align(humidity_label, LV_ALIGN_RIGHT_MID, -30, -30);
humidity_value_label = lv_label_create(lv_screen_active());
lv_obj_set_style_text_font(humidity_value_label, &lv_font_montserrat_30, 0);
lv_label_set_text(humidity_value_label, "--%");
lv_obj_align(humidity_value_label, LV_ALIGN_RIGHT_MID, -30, 10);
// Bouton toggle pour le thème
lv_obj_t * btn = lv_btn_create(lv_screen_active());
lv_obj_add_event_cb(btn, event_handler_btn, LV_EVENT_ALL, NULL);
lv_obj_align(btn, LV_ALIGN_BOTTOM_MID, 0, -15);
lv_obj_add_flag(btn, LV_OBJ_FLAG_CHECKABLE);
lv_obj_set_height(btn, LV_SIZE_CONTENT);
lv_obj_t * btn_label = lv_label_create(btn);
lv_label_set_text(btn_label, "Mode");
lv_obj_center(btn_label);
}
void setup() {
Serial.begin(115200);
dht.begin();
lv_init();
touchscreenSPI.begin(XPT2046_CLK, XPT2046_MISO, XPT2046_MOSI, XPT2046_CS);
touchscreen.begin(touchscreenSPI);
touchscreen.setRotation(2);
lv_display_t * disp;
disp = lv_tft_espi_create(SCREEN_WIDTH, SCREEN_HEIGHT, draw_buf, sizeof(draw_buf));
lv_display_set_rotation(disp, LV_DISPLAY_ROTATION_270);
lv_indev_t * indev = lv_indev_create();
lv_indev_set_type(indev, LV_INDEV_TYPE_POINTER);
lv_indev_set_read_cb(indev, touchscreen_read);
lv_create_main_gui();
updateDHT11();
}
void loop() {
lv_task_handler();
lv_tick_inc(5);
static uint32_t lastUpdate = 0;
uint32_t currentMillis = millis();
if(currentMillis - lastUpdate > 2000) {
updateDHT11();
lastUpdate = currentMillis;
}
delay(5);
}
Le code comprend maintenant :
- Une interface simple en deux colonnes (température et humidité)
- Un bouton toggle pour basculer entre mode clair et sombre
- Une mise à jour toutes les 2 secondes des données du DHT11
- Des icônes appropriées pour la température (°) et l’humidité (goutte d’eau)
- Un affichage des valeurs en grand et des labels en plus petit
- Une gestion du tactile pour le bouton toggle
Les valeurs sont affichées sous ce format :
- Température : avec une décimale (xx.x°C)
- Humidité : sans décimale (xx%)
Vous devriez in fine obtenir ce résultat:
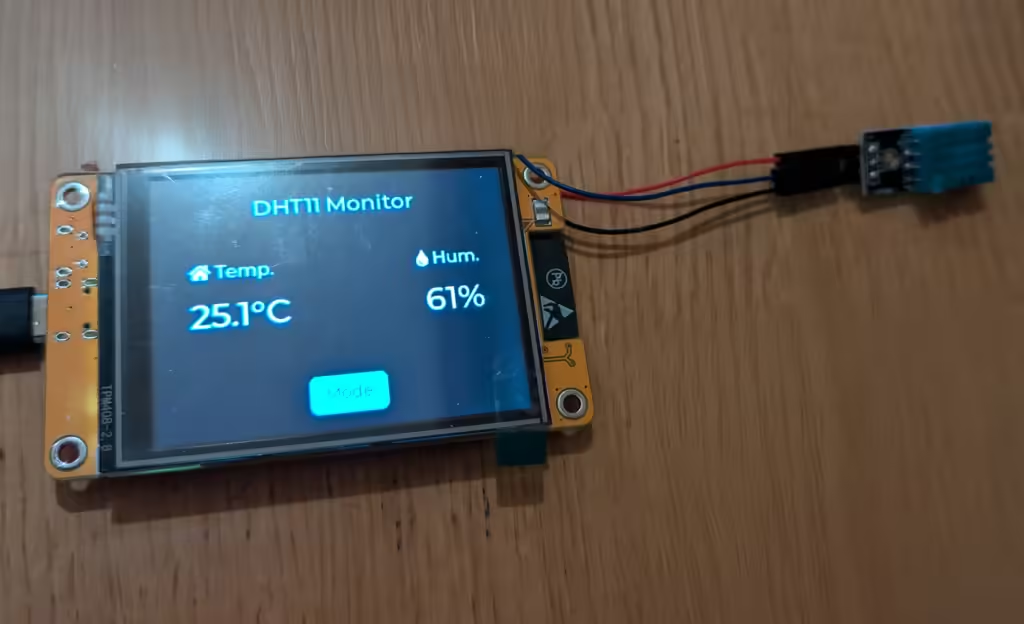